A Developers vision
What is Symfony?
Symfony is a bunch of stand alone PHP libraries
What is Symfony2 Framework?
- A set of bundles containing configuration and bridge classes
- These glue the components together, giving the developer a consistent experience
- Symfony 2 is a PHP Framework for web development
- Symfony 2 is MVC
- Symfony 2 is OOP
- Symfony 2 is not Symfony 1 (>=PHP 5.3)
- Symfony 2 is HTTP-centric
- Symfony 2 is like bits of Rails and like bits of Java
Why Symfony2?
Based on project needs Symfony can be used as:
- Full framework
- Micro framework (single file apps)
- Symfony Components (stand alone)
- Symfony comes with tight integration with many open source projects like Monolog, Assetic, Doctrine, Propel.
- Powerful and easy routing.
- Very advanced and useful template engine TWIG
Symfony2 VS Zend:
- After the launch of Zend framework 2 (ZF2) all the major things offered in Symfony2 are available in ZF2
- Both Symfony2 and ZF2 are the next generation frameworks using PHP 5.3
- Symfony have pre integrated Twig template engine where as now ZF2 comes up with ZfcTwig Modules to support Twig
- Symfony2 and ZF2 are both good for enterprise products
Things You should Know before starting with Symfony2:
- Namespaces in PHP
- YML
- ORM ( Object relationship Model )
- Understanding of design Patterns
- OOP concepts
What can you expect?
22 High quality Components
- DependencyInjection EventDispatcher
- HttpFoundation DomCrawler
- ClassLoader CssSelector
- HttpKernel BrowserKit
- Templating Translation
- SerializerAl Serializer
- Validator Security
- Routing Console
- Process Config
- Finder Locale
- Yaml Form
Highlights of Symfony 2:
- Rewritten from scratch for PHP5.3
- Based on the HTTP specification
- Very stable and solid API ( 12 preview releases, 5 beta releases, 6 release candidates, 9 stable releases )
- Extensible through the creation of Bundles ( replacement for sf1plugins )
- Flexible configuration using YAML, XML, annotations or PHP
- All configuration is compiled to PHP code and cached
- Lots of unit tests
- Source code audited by independent security firm thanks to donations of the Symfony Community
- Extensible Configuration with Service Container / Dependency Injection
- Complete redesign of Forms support
- Validations
- Extensible Security with Authentication / Authorization
- Advanced and powerful templating through Twig
- Routes configured with YAML, XML or Annotations
- ESI Caching support out of the box
- Assets management with Assetic
- Translations
- Environments
What are Bundles?
- Bundle is like a plugin, except that even the core framework is implemented as bundle.
- Your code is an equal citizen with the core
Symfony2 is a set of standalone PHP component libraries, glued together by a group of removable “bundles”
Let's get into some action..
- Download Symfony2 from http://symfony.com/download
- Unzip it into your localhost
- Run the config file http://localhost/Symfony/web/config.php
- This page identifies any problem with your setup
- If you want to configure your setup using GUI then click on “Configure your Symfony Application Online”
- Else you can directly edit Symfony/app/config/parameters.yml
Let's create some pages..
The 3 step approach:
- Request comes in – Symfony matches the URL to a route
- Symfony executes the controller (PHP function) of the route
- The controller (your code) returns a Symfony Response object
Famous Hello World:
- Our goal is to create a Hello world like application
In two small steps…
Step 1: Define a route
In our case we are using YML but xml can also be used to define routes
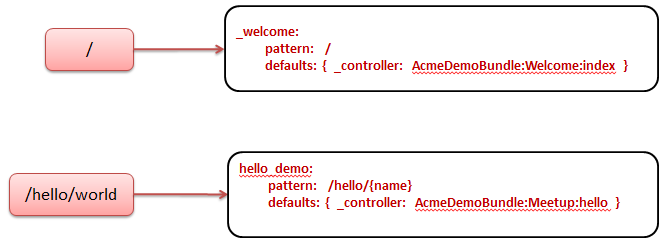
Add the following route to app/config/routing.yml
** Routes can also be defined as XML,PHP and annotations
Step 2: Symfony executes the controller of the route
Step 2: Create the controller
The controller returns a Symfony Response object
Routing Placeholders
Finally!
2 Steps to remember:
- Create a route that points to a controller
- Do anything you want inside the controller, but eventually return a Response object
So far so good….
- Now lets move to templates…
- Why template…?
- Template help you separate your presentation layer from your business logic.
- In a nutshell separate your PHP code and HTML code
Lets Render a template in our controller..
Create the template file..
Still Working
Twig saves a lot of time ..
Learn more about Twig from official documentation: http://twig.sensiolabs.org/
Moving Forward …
Lets create another page to check Ajax and Database calls
Configure database..
All application configuration is placed in “app/config” folder. The one we need to edit is App/config/parameters.yml
Users App
Now we will create Our first form to add users to this table
Step1 : create Your route
Step2: define action in controller
Step3: define template
Step4: create your form
Users App – Step1
Users App – Step 2
Users App – Step 3
Users App – Step 4
Create Form
- For creating the form we will use Symfony2 Form Framework
- Form component in Symfony2 is a very powerful framework that makes the tedious task of dealing with forms easy
- As with all Symfony2 components, it can be used outside of Symfony2 in your own projects
- For using Form component we need to create our Entity, in our case User
- By using the Symfony Form component we create forms that can be re-used in the entire application which leads us to create our form type, in our case UserType
User App Step 4 – create Form -User Entity class
Users App – Step 4 – Create Form – User Form Type class
Users App - Step 4
And its Alive!
Define validations
Save data to database
Symfony2 uses Doctrine2 ORM for Database abstraction
- We will reuse our User Entity class
- Define Database Mapping using Annotation
- Save the record in the database
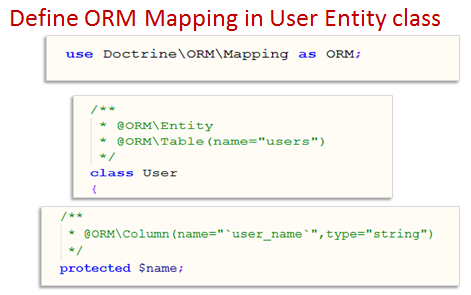
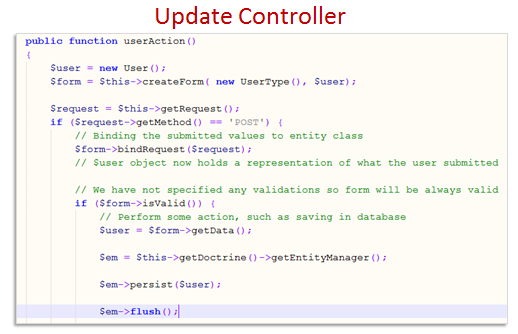
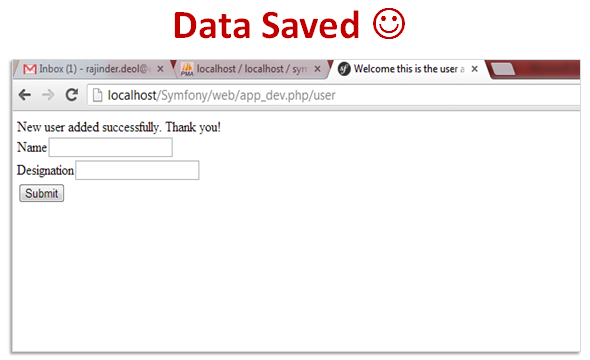
View Saved Users
We will now create a new page to show saved users
Step 1: create route
Step2: Create action
Step3: Create template
Create Route
Create Action
Create Template
Add Navigation and Style
Much Better